TypeScript+React
Event Props
코딩 화이팅
2023. 8. 30. 17:36
OnClick Event Props를 주고 받으면서 적용하기
Button.tsx
type ButtonProps = {
handleClick: (event: React.MouseEvent<HTMLButtonElement>, id: number) => void;
// 이벤트 props를 받기 위해 event: React.MouseEvent<HTMLButtonElement>를 넣고, id: number 받을게 무엇인지
// 넣는다.
};
export const Button = (props: ButtonProps) => {
return <button onClick={event => props.handleClick(event, 2)}>Click</button>;
// 이벤트를 props.handleClick(event로 받고, 2를 number로 정해준다.
};
App.tsx
import "./App.css";
import { Button } from "./components/Button";
function App() {
return (
<div className="App">
<Button
handleClick={(event, id) => {
// event와 id를 매개변수로 정해주고 props로 보내준다.
console.log("Button clicked", event, id);
}}
/>
</div>
);
}
export default App;
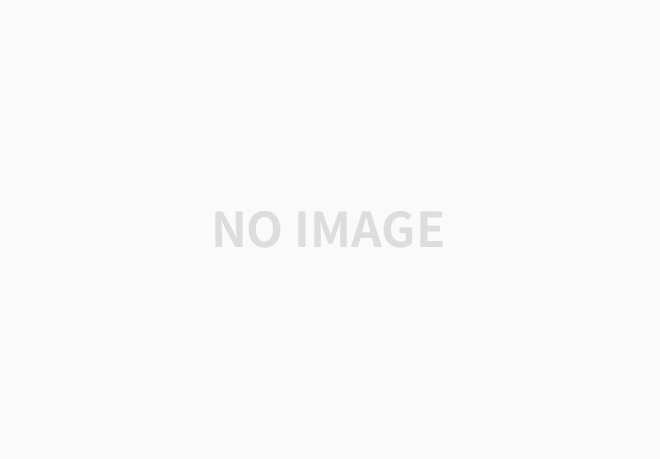
OnChange Event Props를 주고 받으면서 적용하기
Button.tsx
type ButtonProps = {
handleClick: (event: React.MouseEvent<HTMLButtonElement>, id: number) => void;
// 이벤트 props를 받기 위해 event: React.MouseEvent<HTMLButtonElement>를 넣고, id: number 받을게 무엇인지
// 넣는다.
};
export const Button = (props: ButtonProps) => {
return <button onClick={event => props.handleClick(event, 2)}>Click</button>;
// 이벤트를 props.handleClick(event로 받고, 2를 number로 정해준다.
};
App.tsx
import "./App.css";
import { Button } from "./components/Button";
import { Input } from "./components/Input";
function App() {
return (
<div className="App">
<Button
handleClick={(event, id) => {
// event와 id를 매개변수로 정해주고 props로 보내준다.
console.log("Button clicked", event, id);
}}
/>
<Input value="" handleChange={event => console.log(event)} />
</div>
);
}
export default App;
- value prop은 input 요소의 현재 값을 설정
- 사용자가 입력 값을 변경하면 onChange 이벤트 핸들러인 handleChange가 호출
import React, { useState } from "react";
import { Input } from "./components/Input";
function App() {
// 상태를 초기화하여 inputValue에 빈 문자열을 할당
const [inputValue, setInputValue] = useState("");
// 사용자가 입력을 변경할 때 호출되는 함수
const handleInputChange = (event) => {
// 이벤트 객체에서 현재 입력 값을 가져옴
const newValue = event.target.value;
// 상태를 업데이트합니다.
setInputValue(newValue);
};
return (
<div className="App">
<Input
value={inputValue} // 현재 inputValue 상태를 Input 컴포넌트의 value prop로 전달
handleChange={handleInputChange} // 이벤트 핸들러를 Input 컴포넌트의 handleChange prop로 전달
/>
</div>
);
}
export default App;
이런 식으로 value로 props 전달.